年前也就是2013年苗栗三義劉德安的孫子也就是我寫了在C語言實現substr(),沒想到直到如今在Google上搜尋c#substring,會是排名第一的網頁,這真是始料未及的一件事,我猜C#的使用者應該會罵翻這篇文章對他沒有任何用處吧!哥寫C#工作也有三年多時間了,這回應該把我使用C# string Substring的經驗好好分享一下才是,Substring是取得部份字串的方法,底下我們就以Microsoft Visual Studio Professional 2017, .NET Framework 4.7.2為例示範,來回答下面的問題:
- 從字串取得部份字串
- 從字串取得前面n個字元部份字串
- 取得部份字串從指定的 startIndex 處開始, endIndex:到指定的 endIndex處結束
- 取得某個字元後或前的部份字串
- 傳回字串第一次出現位置
- 取得兩字串間的部份字串
- 用字元分離字串再分離字串成部份字串
- C#對中文字串的擷取
要看更多的C#定義及用法可以在微軟官方網站上找到
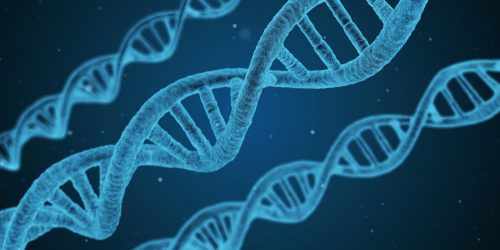
1. C# String.Substring 方法
在C#跟.NET中,字串可以用字串型別來表示,String.Substring方法是C#裡從字串的執行個體擷取部份字串,這個方法有兩個多載的方式:
- Substring(Int32):從字串執行個體擷取部份字串。 部份字串會在指定的字元位置開始並繼續到字串的結尾。
- Substring(Int32, Int32):從字串執行個體擷取部份字串。 部份字串起始於指定的字元位置,並且具有指定的長度。
2.從字串取得前面n個字元部份字串
字串的字元是從0開始索引的,字串第一個字元的位置是從0開始的。
假設你要從字串中取得前面12個字元的部份字串,可以使用Substring方法傳遞開始的索引0跟12的長度,就可以從字串取得前面12個字元的部份字串。
程式片段:
// A long string string bio = "Mahesh Chand is a founder of C# Corner. Mahesh is also an author, speaker, and software architect. Mahesh founded C# Corner in 2000."; // Get first 12 characters substring from a string string authorName = bio.Substring(0, 12); Console.WriteLine(authorName);
現在如果要取得第12個位置後的部份字串,可以傳這個12做為開始的位置參數,看看下面的程式片段:
// Get everything else after 12th position string authorBio = bio.Substring(12); Console.WriteLine(authorBio);
下面是完整的程式片段:
using System; class Program { static void Main(string[] args) { // A long string string bio = "Mahesh Chand is a founder of C# Corner. Mahesh is also an author, " + "speaker, and software architect. Mahesh founded C# Corner in 2000."; // Get first 12 characters substring from a string string authorName = bio.Substring(0, 12); Console.WriteLine(authorName); // Get everything else after 12th position string authorBio = bio.Substring(12); Console.WriteLine(authorBio); Console.ReadKey(); } }
3.取得部份字串從指定的 startIndex 處開始, endIndex:到指定的 endIndex處結束
Substring方法的第一個參數是部份字串開始的索引,第二個參數是包括空白字元在內的字元數,可以使用String. Length找出字串結束的位置。
下面的範利用來找第八個位置到最後的部份字串。
// Get a string between a start index and end index string str = "How to find a substring in a string"; int startIndex = 7; int endIndex = str.Length - 7; string title = str.Substring(startIndex, endIndex); Console.WriteLine(title);
你也可以透過Substring的第二參數來取得一些字元,下面的範例可以從第八個位置取得15個字元的字串。
// Get next 15 characters from starting index string title15 = str.Substring(startIndex, 15);
結果如下:
4.取得某個字元後或前的部份字串
使用Substring取得第一次出現的指定字元前的部份字串,可以透過第一個參數為0,第二個參數為指定字元的位置(長度)來做。
參考下面的程式碼:
// Get a substring after or before a character string authors = "Mahesh Chand, Henry He, Chris Love, Raj Beniwal, Praveen Kumar"; string stringBeforeChar = authors.Substring(0, authors.IndexOf(",")); Console.WriteLine(stringBeforeChar);
下面的程式是取得指定字元後的部份字串:
// Get a substring after a character string stringAfterChar = authors.Substring(authors.IndexOf(",") + 2); Console.WriteLine(stringAfterChar);
結果如下:
5.傳回字串第一次出現位置
使用String.IndexOf方法找出字串中部份字串的位置,這是c# 搜尋字串常用的方法,程式範例如下:
int firstStringPosition = authors.IndexOf(“Henry”);
6.取得兩字串間的部份字串
使用Substring找出兩字串之間的部份字串,第一步要分別找出這兩個字串的位置,再來使用第一個字串的位置做第一個參數,第二個參數以第一個位置到第二個位置的長度來傳遞。
下面範例是找出‘Henry’ 跟 ‘Beniwal’之間的字串:
// Get a substring after or before a character string authors = "Mahesh Chand, Henry He, Chris Love, Raj Beniwal, Praveen Kumar"; // Get a substring between two strings int firstStringPosition = authors.IndexOf("Henry"); int secondStringPosition = authors.IndexOf("Beniwal"); string stringBetweenTwoStrings = authors.Substring(firstStringPosition, secondStringPosition - firstStringPosition + 7); Console.WriteLine(stringBetweenTwoStrings);
結果:
這個部份字串會包括‘Henry’ 跟 ‘Beniwal’這兩個字串,你可以試著增加第一個參數的位置及縮短第二個參數的值來調整。
7.用字元分離字串再分離字串成部份字串
使用 String.Split方法來分隔字串成字串陣列,你可以進一步找出字串的一個字元,再取出一個部份字串。
下面範例會使用’,’來分隔字串,之後再用”:”,來取出部份字串:
// Extract value of each attribute string author = "Name: Mahesh Chand, Book: C# Programming, Publisher: C# Corner, Year: 2020"; string[] authorInfo = author.Split(‘,’); foreach (string info in authorInfo) { Console.WriteLine(" {0}", info.Substring(info.IndexOf(": ") + 1)); }
結果:
以上範例取自Substring in C#
8.C#對中文字串的擷取
C#對中文字串的擷取,在C#中,Substring是最常用的字串擷取函式,但是這種擷取通常一個中文字元只按一個位置計算。
以下範例引用自帶中文的字串擷取
比如:
“我是Lenmong楊”
擷取5個字元就是:
“我是Len”
但其實,我這裡想要的是:
“我是L”
在C#中有很多辦法可以做到,介紹一種最簡便的方法,利用 System.Text.Encoding.Default 的 GetBytes 函式和 GetString 函式。
private string SubStrByByte(string str, int start, int length) { int len = length; int byteCount = System.Text.Encoding.Default.GetByteCount(str); //修改最大長度,防止溢位 if (len > byteCount) { len = byteCount; } var strBytes = System.Text.Encoding.Default.GetBytes(str); string substr = System.Text.Encoding.Default.GetString(strBytes, start, len); //對於半個中文字元的特殊處理 if (substr.EndsWith("?")) { //判斷原字串是否包含問號 var c = str.Substring(substr.Length - 1, 1); if (!c.Equals("?")) { substr = substr.Substring(0, substr.Length - 1); } } return substr + "..."; }
這種中文字串的長度使用(String.Length 屬性)及擷取似乎騙過很多人,我的同事在做考績表的表單程式時,也誤判了這個長度,導致使用者一致輸入錯誤的長度,跟SQL儲存欄位有牽扯時,更容易被搞混。在微軟的String.Length 屬性網頁中,有特別備註說明,列舉於下給各位參考:
備註
Length屬性 Char 會傳回這個實例中的物件數目,而不是 Unicode 字元數。 原因是 Unicode 字元可能會以一個以上的字元表示 Char 。 使用 System.Globalization.StringInfo 類別來處理每個 Unicode 字元,而不是每個字元 Char 。
在某些語言中,例如 C 和 c + +,null 字元表示字串的結尾。 在 .NET 中,null 字元可以內嵌在字串中。 當字串包含一或多個 null 字元時,它們會包含在總字串的長度中。 例如,在下列字串中,子字串 “abc” 和 “def” 會以 null 字元分隔。 Length屬性會傳回7,表示它包含六個字母字元以及 null 字元。
string characters = "abcu0000def"; Console.WriteLine(characters.Length); // Displays 7
※2022/04/08 後記 c# contains用法
可以參考最近翻譯的c# contains用法及程式碼範例
大叔最新分享C# List 定義及七種常用方法,這篇C#教學裡文章裡的contains可以幫你處理c# string find substring的問題,有需要可以點擊查詢閱讀,如果有建議想要問的,可以直接留言給我!
在SQL中也有SUBSTRING函數可以使用,語法如下:
SUBSTRING ( expression ,start , length )
範例:在MySQL BLOB和TEXT型態這篇文章裡有SUBSTRING的範例可以參考。
※2022/05/10
如果你對這篇c# 教學分享沒有獲得收益,歡迎留言分享讓我們改善,或是繼續看《【C#】3小時初學者教學 |Csharp | C# 教學 | C# 入門 | C++++》這部Youtube影片,看能不能有所幫助。
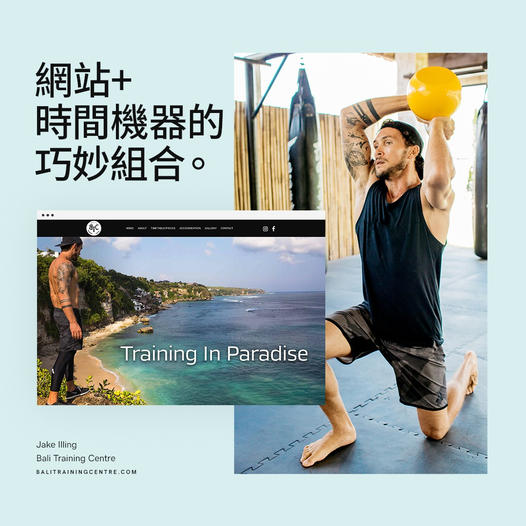
將您的網站交給世界第一的虛擬主機服務。
你現在應該想要架個Windows 虛擬主機,實現絕妙創業構想。立即取得建站神器+行銷,我們將加碼隨附免費網域和 Email。
當然也可以使用免費的Google Sites來架站賺錢,可參閱用G Suite協作平台Google Sites打造專業的賺錢網站在家工作
學 C# 敢找機電整合工程師的工作嗎?
把C#學習列入checklist範本工具使用
※C# List 定義及七種常用方法
※深入淺出物件導向分析與設計
※在C語言實現substr()
2 則留言